Python is one of the most popular programming languages due to its simplicity, readability, and versatility. The true power of Python lies in its extensive ecosystem of libraries, which allow developers to perform a wide variety of tasks, ranging from web development to machine learning and scientific computing. Libraries are prewritten pieces of code that make complex tasks easier and more efficient by providing reusable functions and classes.
In this blog, we will explore a variety of Python libraries categorized by their use cases. Whether you’re a beginner or an advanced developer, knowing the best libraries can significantly enhance your productivity and enable you to develop robust, efficient applications. Let’s dive into the world of Python libraries!
Python Libraries List
1. Data Science and Analytics Libraries
Python has emerged as the go-to language for data science, data analysis, and machine learning because of its extensive libraries. Here are some of the most widely used libraries for data science and analytics:
1.1 NumPy
NumPy (Numerical Python) is the foundation of numerical computing in Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. NumPy is used for performing vectorized operations, and many other data science libraries, like Pandas, depend on NumPy for efficient data handling.
Key Features:
- Fast and efficient array operations
- Supports large, multi-dimensional arrays
- Mathematical functions for linear algebra, Fourier transform, and random number generation
Use case: Efficient handling of numerical data, array manipulation, and performing high-level mathematical operations.
1.2 Pandas
Pandas is a powerful, open-source data analysis and manipulation library. It offers data structures like DataFrame and Series that make it easy to handle and analyze large datasets. Pandas provides built-in functions for handling missing data, data merging, and data reshaping. It is highly compatible with other formats like CSV, Excel, SQL, and JSON.
Key Features:
- Data manipulation with DataFrame and Series
- Handling of missing data
- Grouping, merging, and reshaping of data
- Reading and writing data from various file formats
Use case: Data wrangling, cleaning, and exploratory data analysis (EDA).
1.3 Matplotlib
Matplotlib is a 2D plotting library used for data visualization. It provides a variety of plotting functions to create static, animated, and interactive visualizations. Matplotlib integrates well with NumPy and Pandas, making it easy to visualize data in various formats such as line plots, scatter plots, bar charts, and histograms.
Key Features:
- Create various types of plots (line, bar, histogram, scatter, etc.)
- Customization of colors, labels, and titles
- Integration with other libraries like NumPy and Pandas
Use case: Creating static visualizations for exploring data and presenting results.
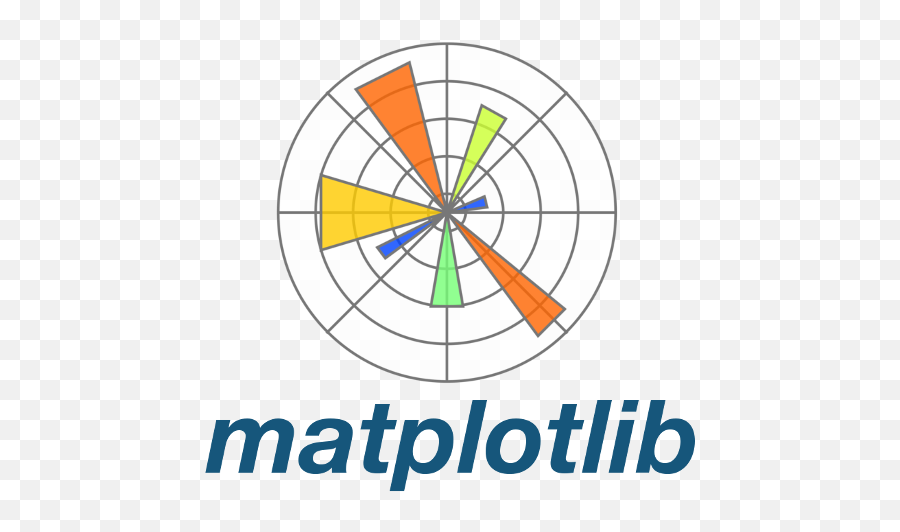
1.4 Seaborn
Seaborn is built on top of Matplotlib and provides an easy-to-use interface for creating beautiful and informative statistical graphics. It comes with several built-in themes and color palettes to enhance the aesthetic quality of the visualizations. Seaborn supports visualizing data distributions, relationships between variables, and statistical analyses.
Key Features:
- Easy-to-use interface for creating complex plots
- Integration with Pandas DataFrames
- Statistical plotting functions (box plots, violin plots, etc.)
Use case: Statistical data visualization, exploring distributions, relationships, and trends in data.
1.5 SciPy
SciPy is a library used for scientific and technical computing. Built on top of NumPy, it adds a collection of algorithms and mathematical tools for optimization, integration, interpolation, eigenvalue problems, and more. SciPy is highly optimized for scientific computing and used extensively in academia and industry for research.
Key Features:
- Optimization and root-finding
- Signal processing, Fourier transform, and filtering
- Integration of differential equations
Use case: Solving mathematical problems, scientific simulations, and numerical optimization.
2. Machine Learning Libraries
Python is one of the leading languages in the machine learning domain. Its flexibility and extensive libraries allow developers to build complex models for tasks like classification, regression, clustering, and reinforcement learning. Let’s explore some of the most popular machine learning libraries:
2.1 Scikit-learn
Scikit-learn is one of the most widely-used libraries for machine learning. It offers a range of simple and efficient tools for data mining and data analysis, and it supports both supervised and unsupervised learning. Scikit-learn includes a variety of algorithms for classification, regression, clustering, and dimensionality reduction.
Key Features:
- Classification and regression models (e.g., decision trees, logistic regression)
- Clustering algorithms (e.g., K-means, DBSCAN)
- Model evaluation and tuning
- Built-in preprocessing tools for scaling, normalizing, and encoding data
Use case: Supervised and unsupervised learning tasks, feature selection, and model evaluation.
2.2 TensorFlow
TensorFlow is an open-source deep learning framework developed by Google. It allows developers to build machine learning and deep learning models, particularly for neural networks. TensorFlow is used for tasks such as image recognition, language processing, and time-series forecasting. TensorFlow is highly scalable and efficient, making it a top choice for both researchers and developers.
Key Features:
- High-level API for building and training models (e.g., Keras)
- Optimized for performance with GPU support
- Integration with other libraries and tools like TensorFlow Lite for mobile and TensorFlow.js for browser-based applications
Use case: Deep learning, neural networks, and machine learning models that require high computational power.
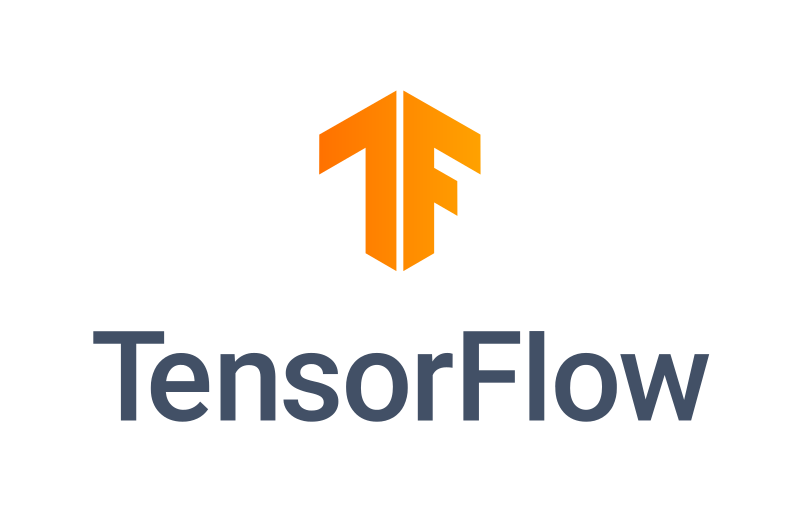
2.3 Keras
Keras is a high-level deep learning library that runs on top of TensorFlow. It simplifies the process of building and training neural networks with its user-friendly interface. Keras is often used for rapid prototyping and experimentation due to its intuitive syntax and integration with TensorFlow.
Key Features:
- Easy-to-use API for building deep learning models
- Seamless integration with TensorFlow
- Support for Convolutional Neural Networks (CNNs) and Recurrent Neural Networks (RNNs)
Use case: Building deep learning models with ease, especially for beginners.
2.4 PyTorch
PyTorch is another deep learning framework that is widely used for machine learning and artificial intelligence research. Developed by Facebook, it is known for its flexibility and speed, allowing developers to build dynamic computation graphs. PyTorch is often favored by researchers due to its ease of use and its strong support for experimentation.
Key Features:
- Dynamic computation graph (eager execution)
- Integration with Python’s scientific stack (e.g., NumPy, SciPy)
- Support for GPU acceleration
Use case: Deep learning, neural networks, and research in AI.
2.5 XGBoost
XGBoost is a highly efficient library used for supervised learning problems like classification and regression. It is particularly known for its performance in Kaggle competitions and is a go-to library for handling structured/tabular data. XGBoost is an implementation of gradient boosting, a machine learning technique that builds strong predictive models by combining the predictions of multiple weak models.
Key Features:
- High performance and speed
- Supports both regression and classification tasks
- Regularization to prevent overfitting
Use case: Classification, regression, and machine learning tasks involving structured data.
3. Web Development Libraries
Python’s ease of use extends to web development, thanks to several libraries and frameworks that simplify the process of building dynamic web applications. Here are some popular Python libraries for web development:
3.1 Django
Django is a high-level web framework designed for building robust and scalable web applications. It includes built-in tools for tasks like database management, URL routing, authentication, and templating. Django promotes rapid development by focusing on reusability and clean design.
Key Features:
- Built-in admin interface
- Powerful ORM (Object-Relational Mapping) for database management
- Authentication, URL routing, and middleware support
- Secure and scalable architecture
Use case: Full-stack web development, creating complex, database-driven websites.
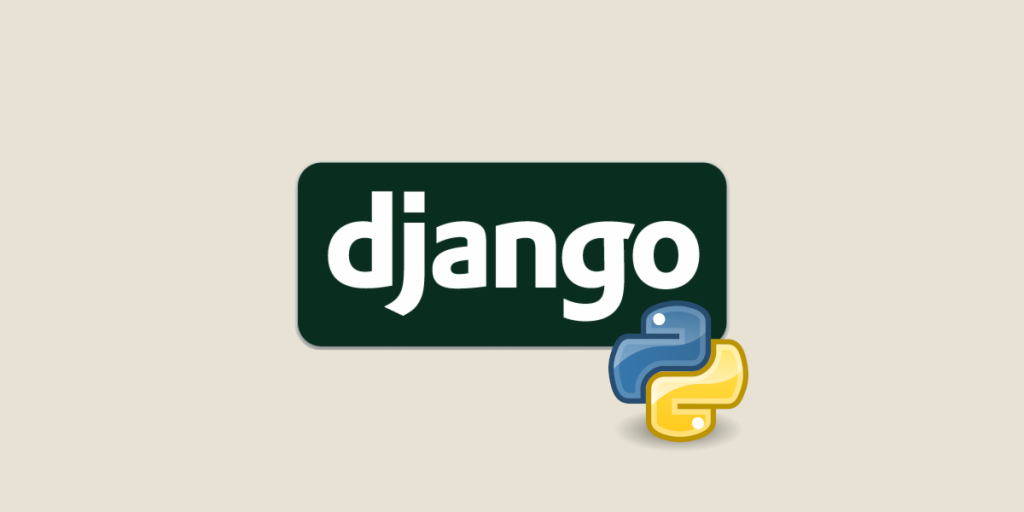
3.2 Flask
Flask is a lightweight, micro-framework for building web applications. Unlike Django, which is full-featured, Flask offers more flexibility by allowing developers to choose their own components. It is ideal for small projects, REST APIs, and microservices.
Key Features:
- Minimalistic framework for building web apps
- Easy to integrate with other tools and libraries
- Extensions for adding features like authentication, database management, and more
Use case: Small-scale web applications, REST APIs, and microservices.
3.3 FastAPI
FastAPI is a modern web framework designed to build high-performance APIs. It is based on Python’s type hints and focuses on rapid development and automatic validation of request data. FastAPI supports asynchronous programming, making it one of the fastest frameworks for API development.
Key Features:
- Fast and efficient (built on top of Starlette and Pydantic)
- Automatic request validation using Python type hints
- Asynchronous programming support for better
performance
Use case: Building high-performance APIs, especially for data-intensive applications.
4. Automation and Web Scraping Libraries
Python is also well-known for its ability to automate repetitive tasks and scrape data from websites. The following libraries make automation and web scraping easy and efficient:
4.1 Selenium
Selenium is a powerful web automation tool. It allows developers to automate browser actions, such as clicking buttons, filling forms, and scraping content from web pages. Selenium supports multiple browsers and is highly used in web scraping and automated testing of web applications.
Key Features:
- Browser automation for testing and web scraping
- Supports multiple browsers (Chrome, Firefox, Safari)
- Integration with testing frameworks like PyTest and Unittest
Use case: Web scraping, browser automation, and web testing.
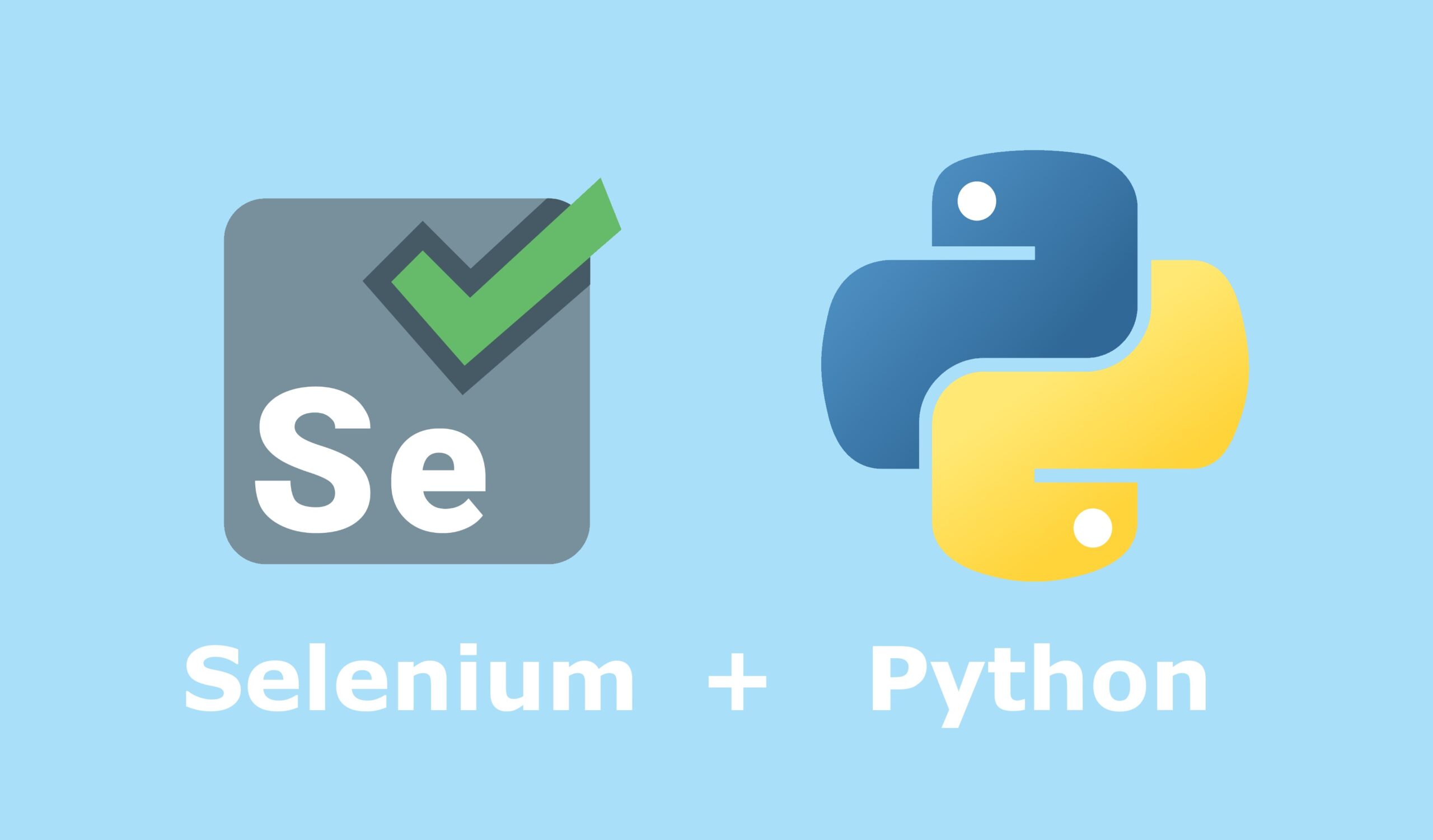
4.2 BeautifulSoup
BeautifulSoup is a Python library used for parsing HTML and XML documents. It allows developers to extract data from web pages by navigating the parse tree and searching for specific elements. BeautifulSoup is often used in conjunction with requests to scrape content from websites.
Key Features:
- HTML and XML parsing
- Easy navigation of the DOM (Document Object Model)
- Integration with requests for scraping web pages
Use case: Web scraping for data extraction and web content analysis.
4.3 Scrapy
Scrapy is a web crawling framework that enables developers to extract structured data from websites. It provides an easy-to-use API for building and running crawlers, which can collect data from multiple web pages efficiently.
Key Features:
- Efficient web scraping framework
- Supports crawling multiple pages
- Handles complex web scraping tasks like handling CAPTCHAs, form submissions, etc.
Use case: Large-scale web scraping, web crawlers for collecting data from various sources.
5. Game Development Libraries
Python is also used in game development, thanks to libraries that provide easy access to game engines and graphical interfaces.
5.1 Pygame
Pygame is a set of Python modules designed for writing video games. It provides functionality for creating 2D games, including tools for handling graphics, sound, and input devices like keyboards and mice.
Key Features:
- 2D graphics and animation
- Audio and sound support
- Input device handling
Use case: Building simple 2D games, learning game development.
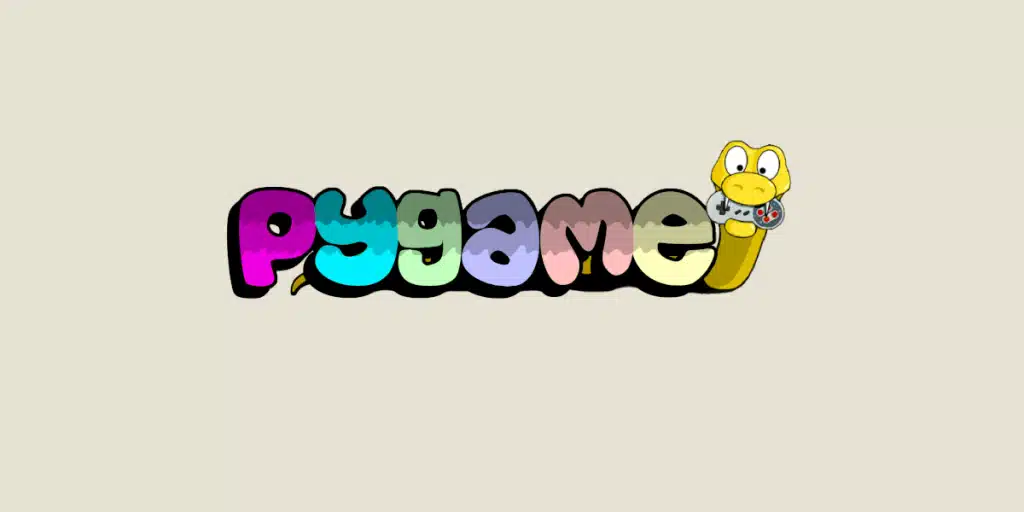
5.2 Panda3D
Panda3D is a game engine and framework for 3D game development using Python and C++. It provides tools for rendering 3D graphics, handling physics, and building interactive 3D environments.
Key Features:
- 3D rendering and physics engine
- Tools for building 3D games and simulations
- Scripting in Python for game logic
Use case: Developing 3D games, interactive simulations.
Conclusion
The Python ecosystem is vast and continuously growing, with a library for almost every task imaginable. From data science and machine learning to web development, automation, and game development, Python libraries have revolutionized the way developers approach problems and build solutions. By mastering these libraries, Python developers can significantly improve their productivity and create more powerful, efficient, and innovative applications.
Whether you’re working with data, building a web app, scraping content from the web, or developing a game, Python’s library ecosystem offers the tools you need to succeed. By understanding and incorporating these libraries into your projects, you’ll be able to unlock the full potential of Python.
The Python Standard Library — Python 3.13.0 documentation – Click Here!!!
More Posts:
Cleaning Kit for Laptop: Keep Your Device Spotless in 5 ways to best
Java 8 Features with Examples – Ultimate Developer Guide for Success
Frequently Asked Questions
How many libraries are there in Python?
What are the top 10 Python libraries?
The top 10 Python libraries, widely used for various tasks, are:
- NumPy – for numerical computations.
- Pandas – for data manipulation and analysis.
- Matplotlib – for data visualization.
- Scikit-learn – for machine learning.
- TensorFlow – for deep learning and neural networks.
- Keras – for building neural networks.
- Flask – for web development (lightweight).
- Django – for full-stack web development.
- Requests – for HTTP requests and web scraping.
- BeautifulSoup – for parsing HTML and web scraping.
These libraries form the core of Python’s versatile ecosystem, supporting data science, machine learning, web development, and more.
What are the Python libraries?
How to install libraries in Python?
To install libraries in Python, you can use pip, Python’s package installer. Open your terminal or command prompt and use the following command:
pip install library_name
For example, to install NumPy, you would run:
pip install numpy
You can also install multiple libraries at once by separating their names with spaces. To install a specific version of a library, use:
pip install library_name==version
To install libraries from a requirements file, use:
pip install -r requirements.txt
Ensure that pip is updated to the latest version using pip install --upgrade pip
.
How to list all the libraries in Python?
To list all installed libraries in Python, you can use the pip
package manager. Open a terminal or command prompt and run the following command:
pip list
This will display a list of all installed Python packages along with their versions. Alternatively, you can use the following code within a Python script or interactive shell:
import pkg_resources
installed_packages = pkg_resources.working_set
for package in installed_packages:
print(package.project_name, package.version)
This will print the names and versions of all installed libraries in your Python environment.